Leverage helper methods for common API endpoints and interact with the editor programmatically.
Developer Toolkit
Welcome Developers
The Design Huddle API is split between two account types: Admins and Users. The Admin API is for the Design Huddle client administrators to leverage admin-only functionality like user and template management, while the User API can be used to build a direct integration with a public-facing website where each request runs in the context of the current user. Both leverage the same OAuth2-based authentication system with the same REST-based structure. To jump directly into the API definitions, use one of the two links below, otherwise continue to the Getting Started section.
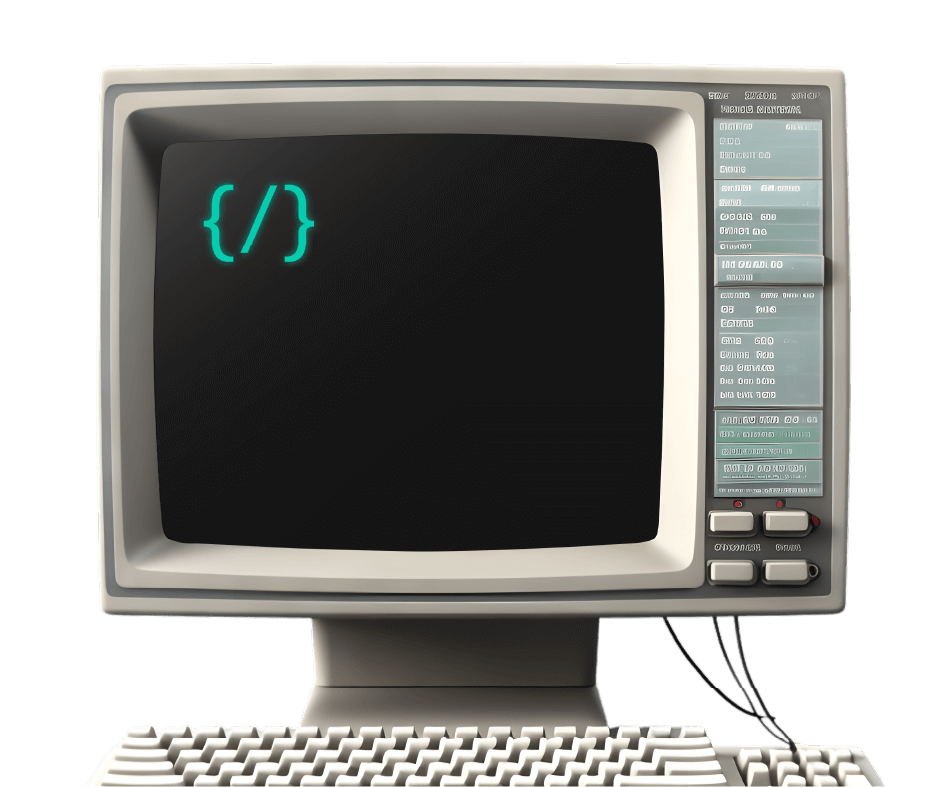
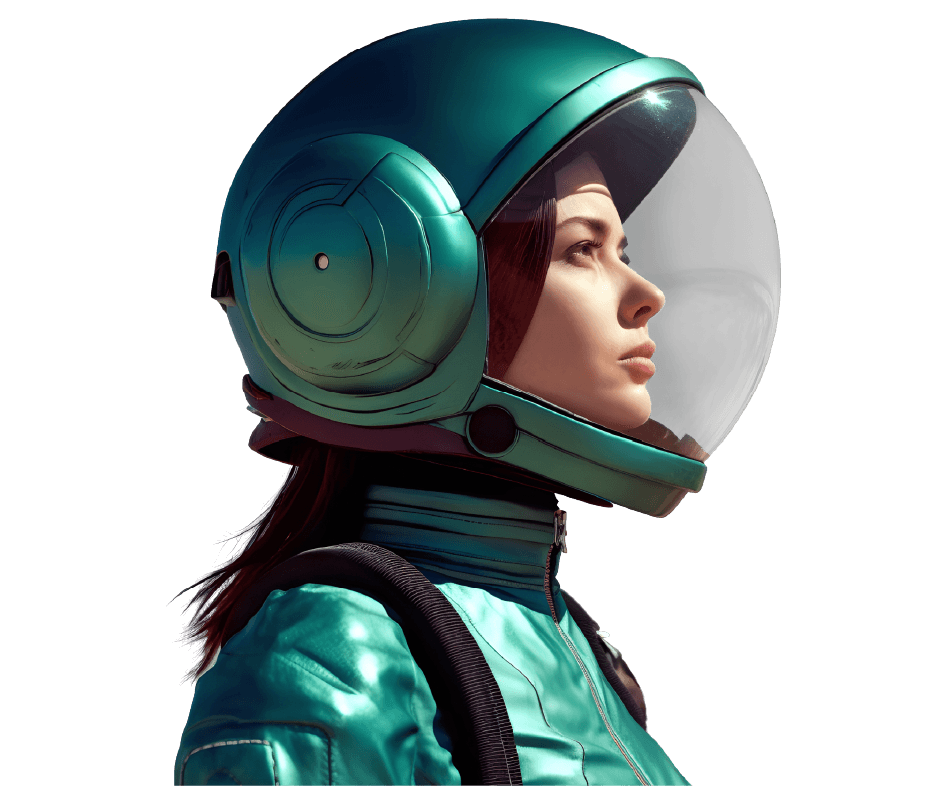
Admin API
Access admin-only functionality like user and template management, reporting and system configuration.
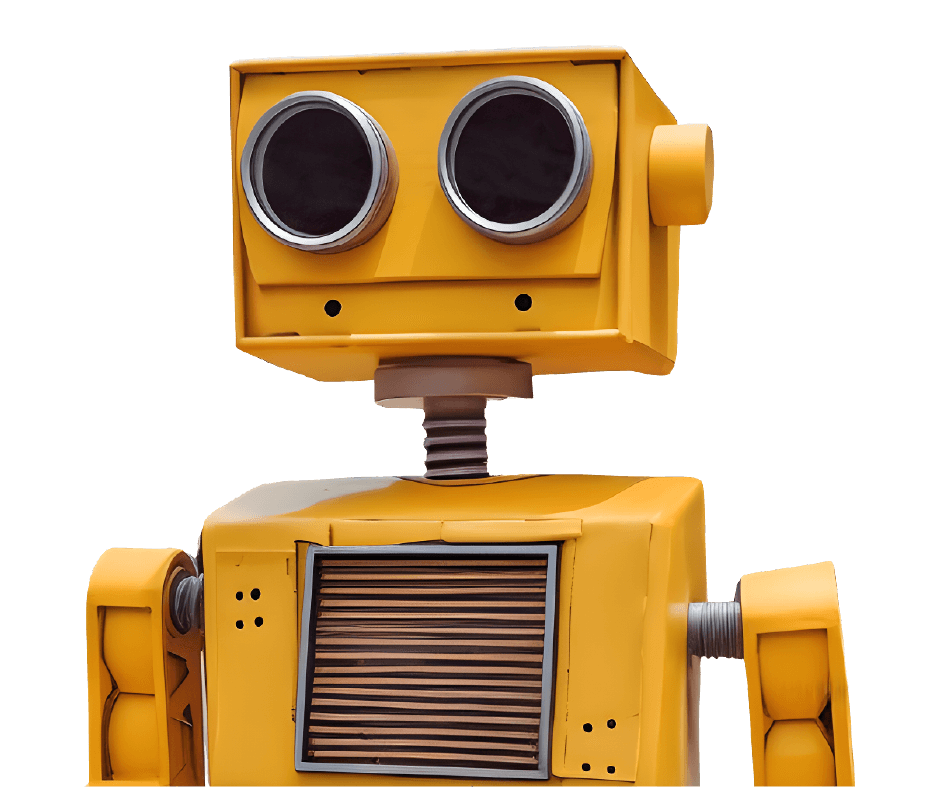
Getting Started
The first step is to create a Private App within the Design Huddle UI. This will provide you with the necessary OAuth2 authentication information to retrieve an Access Token. This token must be used on all subsequent API requests.
You can then test out the various API endpoints via Postman with their respective links throughout the Admin and User API pages, or simply write your own custom REST-based code. Each Design Huddle customer uses their unique (sub)domain for API access, so be sure that is set correctly in any request attempts.
From there you may want to first list the available design templates to retrieve a template id/code and then use that to create a new user-specific project from a template. Or list the user’s existing projects to allow the user to continue designing where they previously left off. Or allow the user to create a new project from scratch, with a canvas size and optional background shape/color/photo. With a project created, you can then launch the embedded editor to allow the user to start designing. Finally you can use the export methods to export the project for print or digital.
Authentication
Authentication for both the Admin and User API is handled via a shared OAuth2-based token endpoint at /oauth/token, accepting the client_id and client_secret obtained from the Private App in the Design Huddle UI. This endpoint returns two possible token types: User Access Tokens and App Access Tokens. While User Access Tokens can be used in requests to any API method, App Access Tokens can only be used on methods in the Admin API that don’t require a user context.
User Access Tokens
User Access Tokens are retrieved by using the Resource Owner Password Credentials Grant Type against the token endpoint (grant_type=password). Design Huddle uses a slightly modified version of this grant type as the username field is required but the password field is not allowed (send in ‘password=1’ if you’re using a client SDK that forces its inclusion). The intention of this method is NOT to pass through (likely insecure) authentication requests from a third party login form, but simply to retrieve a token for a pre-authenticated user, piggy-backing on your own authentication system. As such, CORS is not supported on user access token requests. The username can be either an email address or any other unique identifier.
If you want to create an unauthenticated (Guest) user session, you can use either the Editor Javascript SDK, supplying guest: true in the configuration object to any relevant method, or you can generate a new unique identifier and pass that in as the username to the /oauth/token/guest endpoint. The SDK will automatically cookie the user so it can retrieve their previously created projects (within the guest project expiration timeframe) if/when they return, but if creating Guest Users via the guest token endpoint directly, you would need to manage that process yourself. Note that Guest Users can be "upgraded" to full users if/when they later authenticate on your site.
App Access Tokens
App Access Tokens are retrieved by using the Client Credentials Grant Type (grant_type=client_credentials), and can only be used on specific API methods in the Admin API. As no user is specified, only methods not requiring a user context can be called. In the Admin API documentation, these methods are denoted with both UserAccessToken and AppAccessToken in the Authorizations list.
After receiving an Access Token from the token endpoint, the Bearer Authentication Scheme is used to attach the (bearer) token to the G header of each API method request. All API methods require this header and will return an error if not provided.
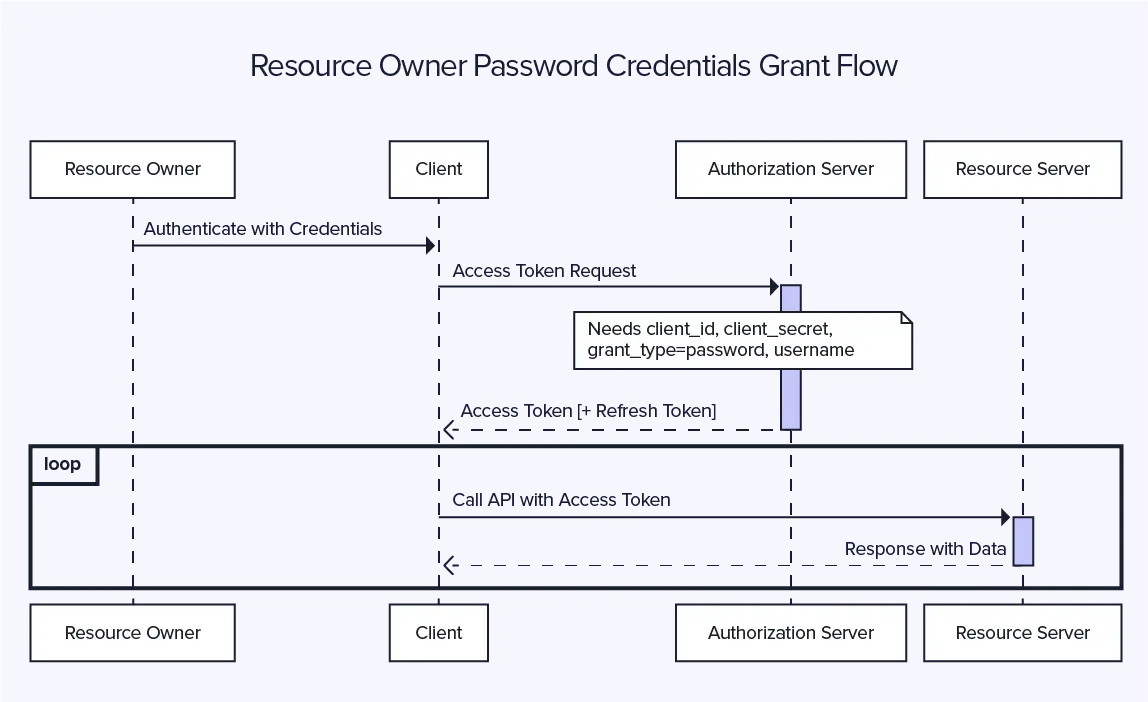
Account/User Synchronization
In addition to the standard OAuth2 input parameters, the /oauth/token endpoint also supports the following (optional) custom parameters that allow for easy synchronization of accounts/users with a separate source database:
email_address | The user’s email address. If not supplied explicitly, this could be inferred from the username field. |
user_code | A custom supplied ID representing the user. If not supplied explicitly, this could be inferred from the username field. |
user_id | The Design Huddle User ID assigned to a previously created user. |
user_status | A status to set the user to. The only value that would make sense here is active which would allow auto-reactivation of a previously disabled user during login. |
first_name | The user’s first name. |
last_name | The user’s last name. |
user_properties | A JSON object representing any other custom properties assigned to this user. |
role_id | One or more Design Huddle Role IDs representing the override permission level this user should be granted. |
account_code | A custom supplied ID representing the account. |
account_id | The Design Huddle Account ID assigned to a previously created account. |
account_status | A status to set the account to. The only value that would make sense here is active which would allow auto-reactivation of a previously disabled account during login. |
account_name | A custom supplied name to refer to the account. |
account_properties | A JSON object representing any other custom properties assigned to this account. |
This endpoint supports full “upsert” functionality. Users are looked up via either their Email Address, User Code or User ID. If found, their existing record will be updated with any new data supplied. If not found, a new user will be created with all supplied data. Note that the username parameter will be interpreted as either the user’s Email Address or User Code (depending on format) assuming those parameters are not explicitly supplied.
In Design Huddle, multiple users can be created under a single account. However if you don’t require multi-user accounts, simply ignore the account properties and manage users directly. The parent account entity is informational only if not maintained intentionally.
ID Mapping
Most entities in Design Huddle have a “code” field representing a third party identifier that you may use to synchronize with your source database. This removes the need for you to add database columns to your database tables as Design Huddle stores your ID’s instead. All of the Design Huddle API methods support entity references by their internal ID or the custom supplied code.
To reference an entity by your ID in a restful API path, simply prefix your ID with “code-“ in place of the internal ID. As an example, if you wanted to update a Template with an internal ID of 23, you would typically submit a PATCH request to /templates/23, however if this template had your identifier “abc” stored as its template_code, you could submit the PATCH request to /templates/code-abc instead.
While POST and PATCH methods are available for entity creation and updates respectively, the PUT method can be used to perform “upserts” when using your custom supplied ID. This removes the need to make an initial GET request to determine if an entity has already been created in Design Huddle before deciding to either insert or update it. Simply submit a PUT request with your ID instead, and Design Huddle will update the entity if it already exists, or create it if not. When taking advantage of this functionality, be sure to always supply all required fields to ensure an entity can be successfully created from scratch if necessary.
Embedding
The editor iframe can be easily and securely embedded into any other website of your choice. First specify which domain(s) to allow in the Private App configuration in the Design Huddle UI. Then use the Editor Javascript SDK or drop a custom iframe on your site with your specific editor URL including the project_id to open and a token in the querystring. The value of the token parameter should be the user-specific access token previously received from the OAuth2 token endpoint. The project_id can be obtained by listing the current user’s existing projects or by calling the Create Project endpoint specifying either a template to customize or the properties for creating a new project from scratch.
Example HTML:
<iframe
id= "editoriframe"
src= "https: //[subdomain].designhuddle.com/editor?project_id=[project_id]&token=[token]"
width= "100%"
height= "100%"
frameborder= "0"
></iframe>
Because a (short-lived) token is passed into the iframe, 3rd party cookies are not necessary for user authorization, which greatly increases compatibility in today’s browsers. Direct API requests from your front-end code use bearer tokens to avoid cookies as well. CORS is also supported on these requests, only allowing origin domains from the domain list you configure in your Private App. Note that CORS is not supported in the token request itself, tokens must first be retreived via backend request so as not to expose the Client ID/Secret publicly.
The Editor Javascript SDK can be used to facilitate back and forth communication between your site and the editor iframe. This enables your site to:
- Retrieve metadata about the open project and all its elements
- Retrieve real-time rendered images of the latest design changes
- Update the current project, injecting new media and altering existing elements
- Override default editor behavior and events
- Set/Replace the Product Image Background in the editor
- Receive notifications of various user triggered events that occur within the editor
- Receive notification of an authorization issue prohibiting the use of the editor
- Receive notification of a network error prohibiting the use of the editor
Errors
In addition to the method-specific errors listed in the Admin and User API Documentation, while very infrequent, the API may also return the following error codes:
401/403
Either your access token has expired or the current user does not have access to the resource requested. Try requesting a new access token first but if this error occurs repeatedly you can look into the role and permissions assigned to this user
404/415
The path or resource requested was not found. Do not retry this request.
429
The system is currently under extreme load. Please retry the request after a few seconds.
500
An unknown error occurred processing your request. If you receive this repeatedly please contact us at support@designhuddle.com.
502/503/504
A network connection issue occurred during the request, please try again after a few seconds. If you receive this repeatedly please contact us at support@designhuddle.com.